Learn how to build a DApp have 2 main parts. The 1st part is to learn how to write a smart contract and deploy it on a blockchain network. The 2nd part is to learn how to interact with data and smart contracts on the blockchain network.
Introduction
We learn some basic ideas to write a token smart contract on the tutorial “Issue Your Own Token – Ethereum for Beginners“. In the tutorial, we also learn how to use remix IDE, Metamask wallet and etherscan to interact with Ethereum blockchain or retrieve data from Ethereum blockchain. The fundamental of Metamask wallet and etherscan is web3.js.
Web3.js is the most popular js library to interact with Ethereum blockchain. There are some steps you can get started with web3.js.
- Read your Ethereum account balance through web3.js.
- Make balance transactions on the Ethereum network through web3.js.
- Make deploying smart contract transactions on Ethereum through web3.js.
- Interact with your smart contract through web3.js.
Environment
Before you get started, you may need to set up some environments.
Node.js is a program to let you execute your web3.js and other js applications. Npm is Nodejs package manager, you will install your web3.js and other packages through npm.
2. Ethereum node
You may need to prepare an Ethereum node to interact with. When we say “interact with the Ethereum network”, most time we mean to interact with an Ethereum node. When the node synchronizes with the public blockchain network, you can interact with the blockchain network.
There several ways to prepare an Ethereum node to interact with.
- Use Ganache. Ganache helps you to build a local node very quickly for you to get start. However, it spends time synchronizing with the public network.
- Use go-ethereum or parity. These are the two most popular Ethereum client applications. However, it takes time if you want to get a full node on your client.
- We suggest you use Infura here. Infura helps to run a full node for you to interact with, you don’t need to spend hours to download an Ethereum node.
Get Start – Check Balance
Make sure you already install Node.js and NPM. Open your terminal in an empty folder, and sent
npm install web3
You will see a file named “package.json” and a folder named “node_modules” been created. Your web3.js package is in the node_modules folder now. The dependency of packages is written on “package.json”.
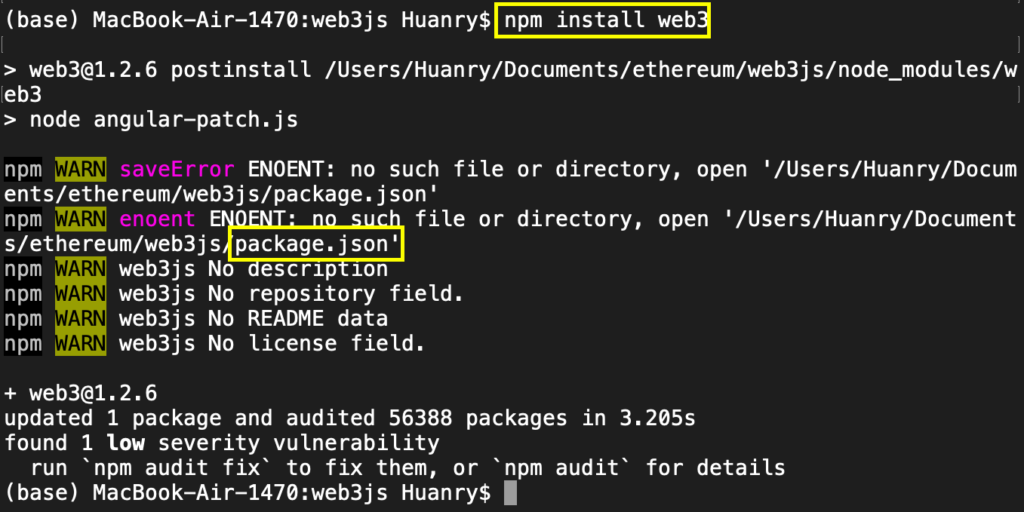
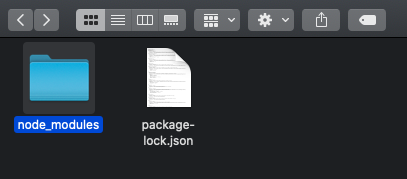
Now, write a file named “app.js” in the same folder
const Web3 = require(‘web3’)
const web3 = new Web3(‘Your Node Endpoint ‘)
web3.eth.getBalance(‘Your Account Adress‘, (err, wei) => { balance = web3.utils.fromWei(wei, ‘ether’); console.log(“I have ” + balance + “ETH on Ropsten Network”) })
Replace “Your Node Endpoint” and “Your Account Adress“. You should get your endpoint on your Infura.io dashboard.
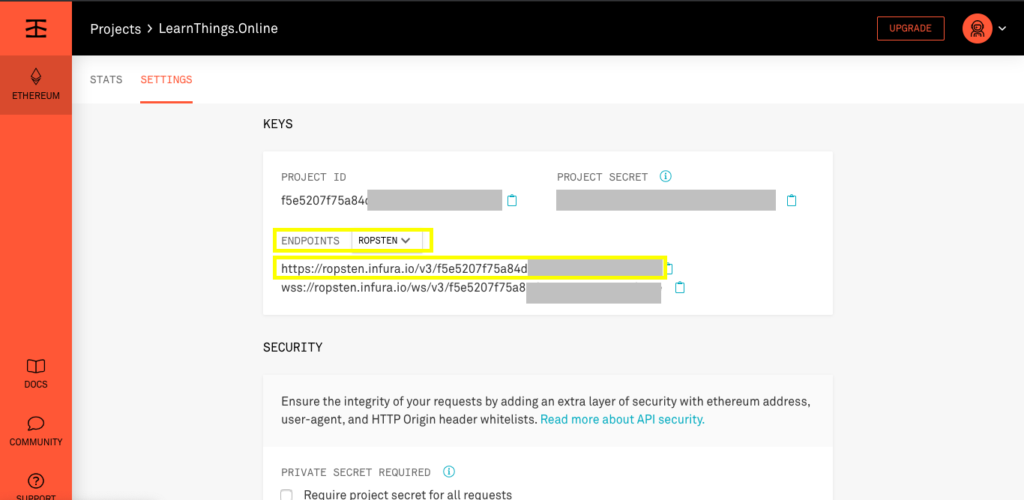
Now, run your application by
node app.js
You can check how many ETH in your account now.

Go Further – Make Transaction
To make a transaction, we add two packages.
npm install ethereumjs-tx dotenv –save
ethereumjs-tx is an Ethereum VM implemented in Javascript, it helps you to make transactions on Ethereum. dotenv is a package that helps you to store some informations separately. (You don’t need this package, but it can help you to protect your private key.)
Create a file named “.env”, write some informations you want to protect into the file.

Make another file named “app2.js”
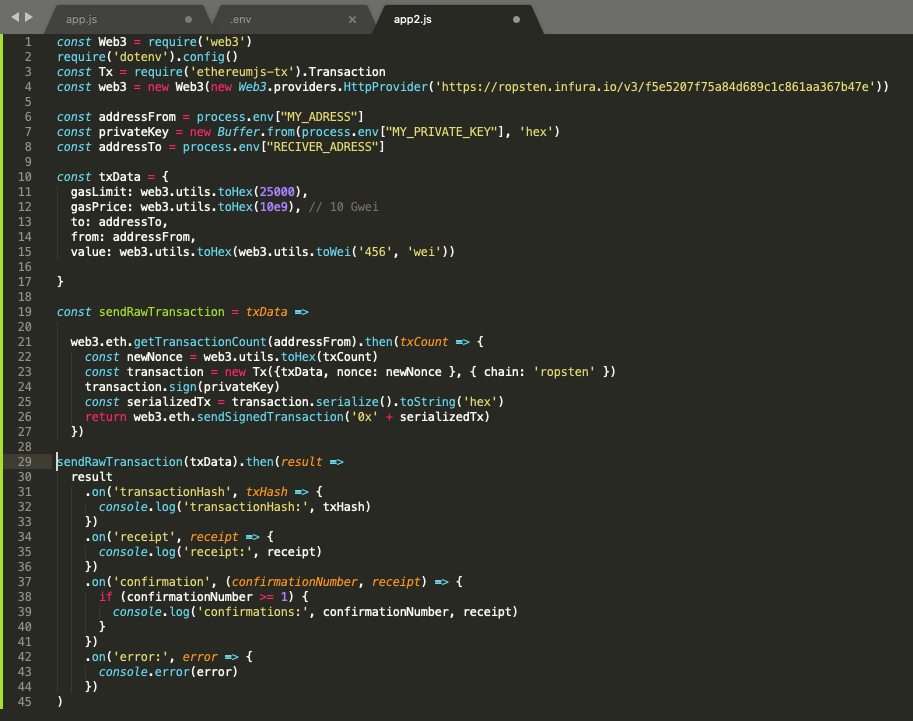
We copy the code from Github (raineorshine/sendRawTransaction.js) We try to separate account informations to “.env” file. Check lines 2, 6, 7, 8 to check how to do it.
For line 15, you can set how much you like to sent to the receiver.
You can make the transaction now.
node app2.js
You can check the transaction on etherscan.

You made your first two applications to interact with Ethereum using web3.js now. (The first step in the introduction.) You can try to image others you can do through web3.js.
To learn more about web3.js, check “Read More” (We may also write more on LearnThings.Online, keep check on our website.)
Read More
Ethereum Dapp for Beginners – Full Stack Hello World Voting Ethereum Dapp Tutorial (You can find online resources to learn how to deploy a smart contract on Ethereum and interact with it through web3.js)
Mastering Ethereum: Building Smart Contracts and Apps (A good book to learn Ethereum, written by Andreas M. Antonopoulos and Gavin Wood. Gavin Wood is the cofounder of Ethereum who also found Party and Polkadot)
Books for Ethereum development on Amazon
Why users still make use of to read news papers when in this technological globe the whole thing is
accessible on net?