One of the most popular applications of Ethereum is to issue your own cryptocurrency. In this tutorial, we’ll teach you how to issue your own cryptocurrency without/with code.
Before we start, we need to understand there are different types of cryptocurrency.
One is cryptocurrency in the consensus system of one blockchain network. For example, BTC in the bitcoin network, ETH in the Ethereum network. In this case, people first get the currency from a process called mining. Through the mining process, miners(or nodes) will get incentives to support the infrastructure of the blockchain network. All mechanism for the incentive is written in the code for the blockchain. People in the mining process support the price or value of the currency.
Another one is the token contract on the top of a blockchain. The most popular case is ERC20 token on Ethereum. You can deploy a token smart contract on a blockchain to issue your own token. It cost you just a little to deploy the contract on the blockchain. If you didn’t do other things to support the value of your own token, people may say it’s air-coin or shit-coin.
ERC20 is the most popular standard to issue your “contract token”. People often use ERC20 to issue their own token for their ICO for crowdfunding.
ie. ICO stands for “initial coin offering”, some blockchain project sell their coin before they build their project. Then build the project to interact with the coin.
Now, we can start.
Issue your own token without code
Pre-request: you need to have Ethereum-wallet, we suggest you use Chrome Extensions Metamask. Also you need to have ETH in your account.
In this tutorial, we use the “Ropsten Test Net”, you can get your “fake ETH” through https://faucet.ropsten.be
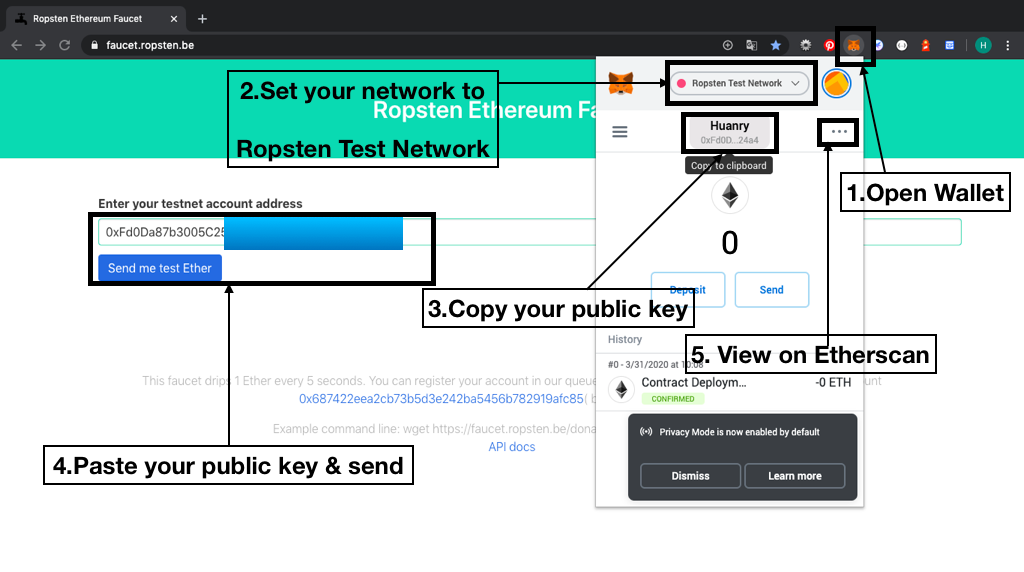
You can see “ETH” on Etherscan and your wallet.
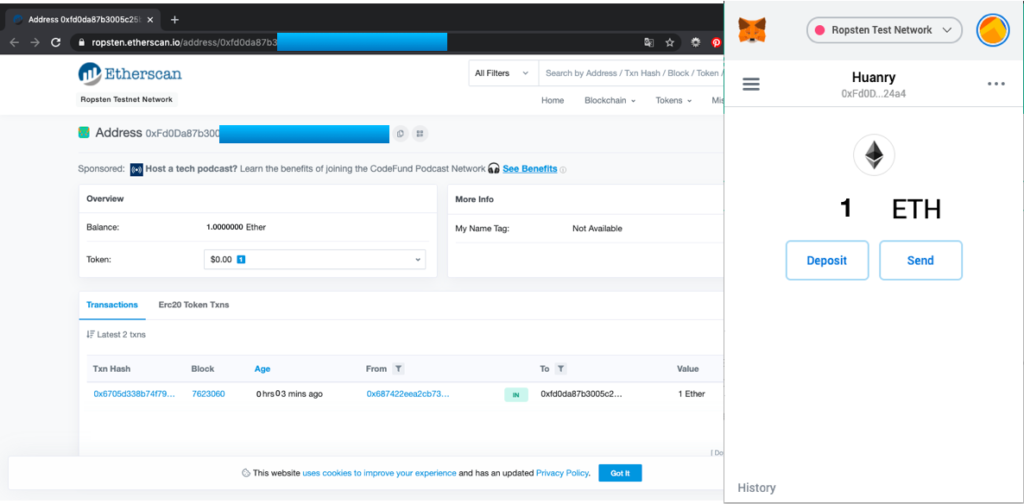
Go to the ERC-20 generator to type
https://vittominacori.github.io/erc20-generator
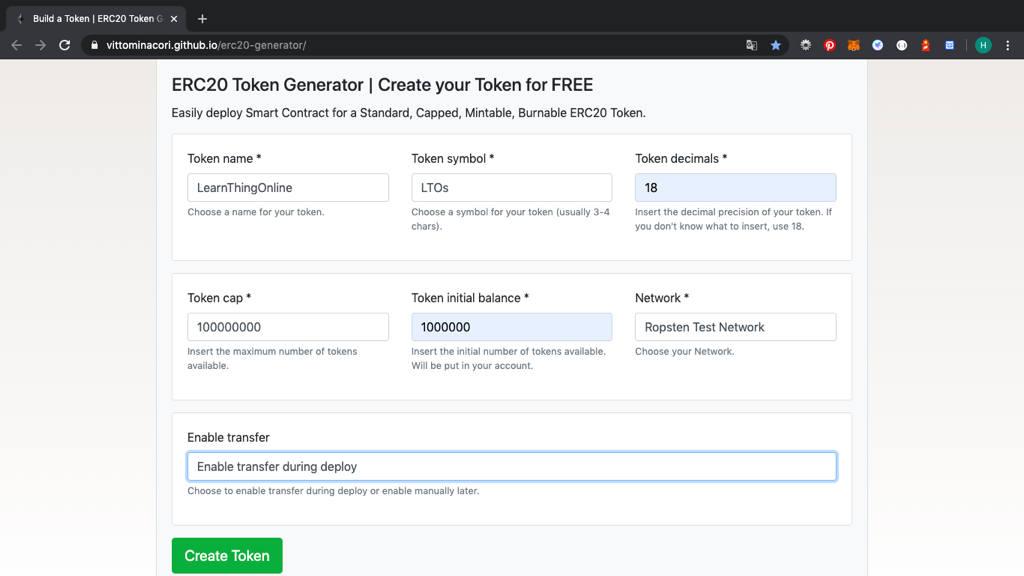
You can see the wallet will ask you to pay the “gas fee” by ETH to deploy the contract.
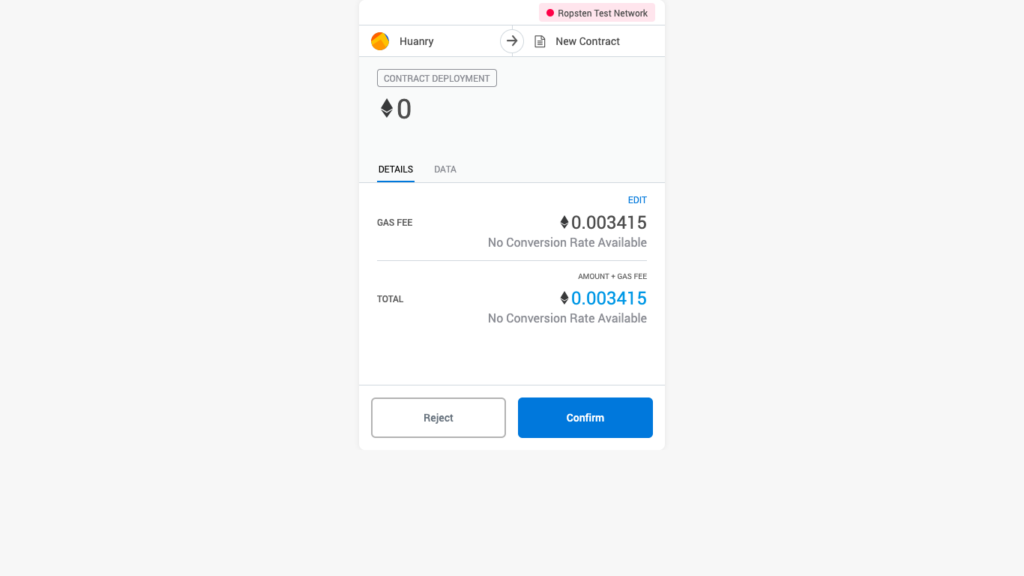
It’s down, you can see your smart contract address, click to check information on etherscan
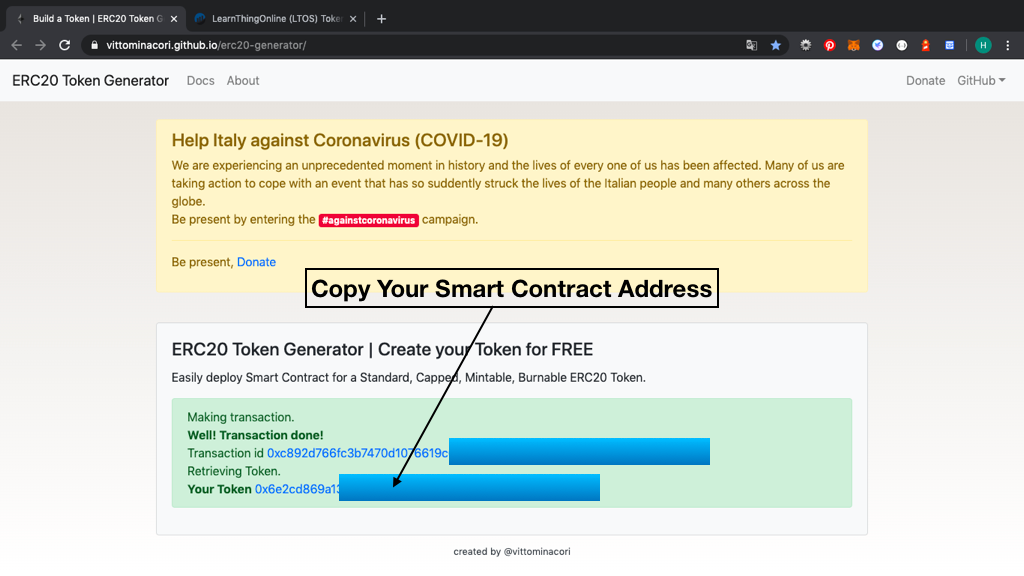
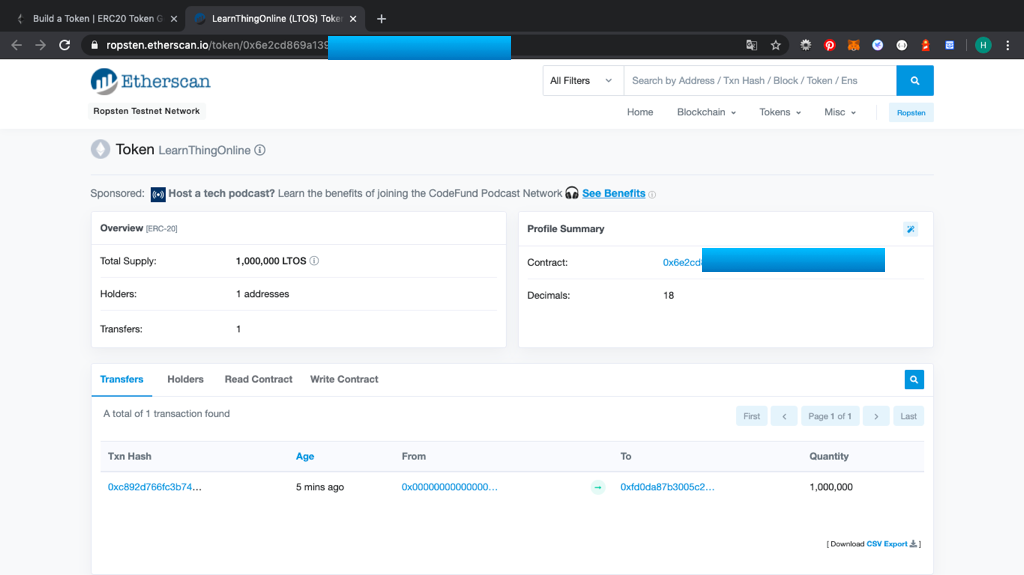
Also, you can check the etherscan with your wallet address
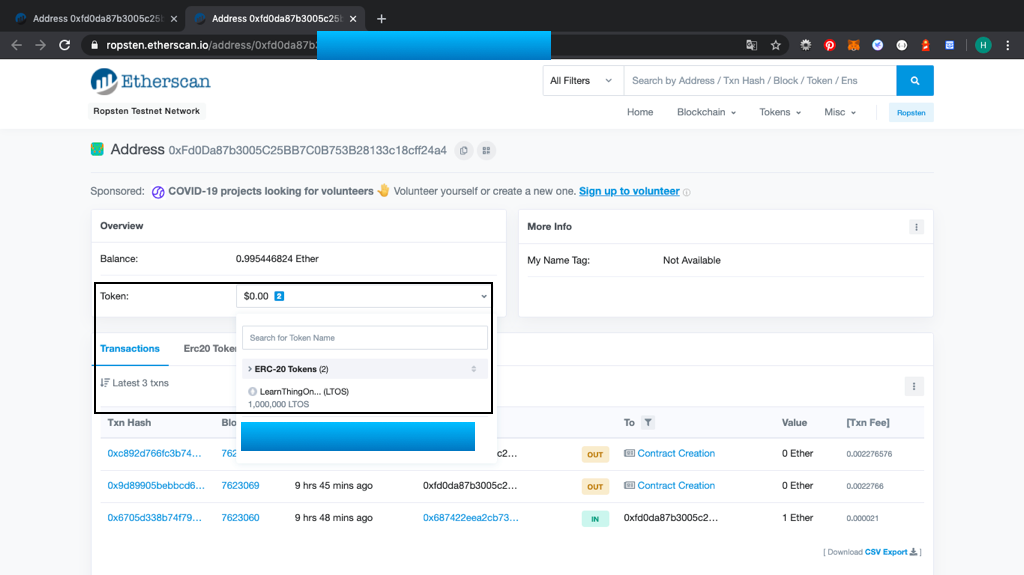
If you want to send your token to others, you can add the token on your wallet.
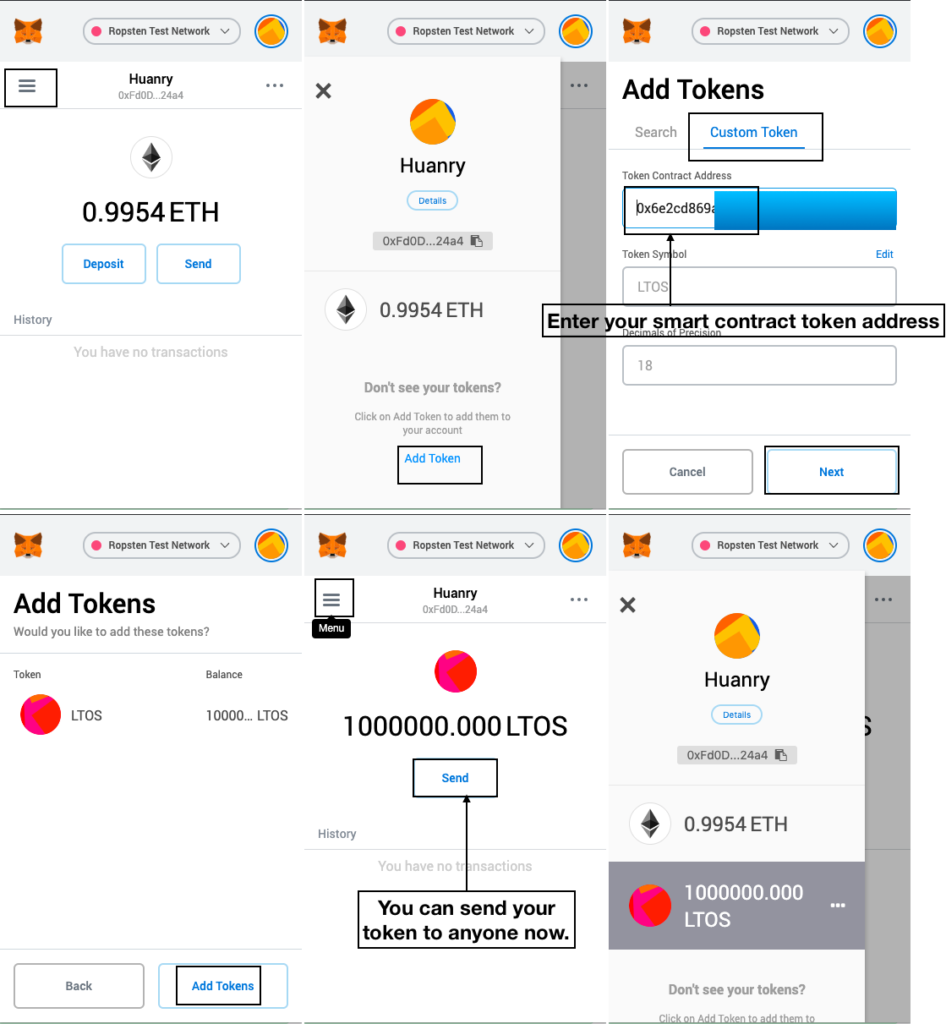
Congratulations! You have your own token now.
Let’s review the process to get the token.
- Type properties we like for the token. (The ERC-20 generator actually helps you to put these parameters on the smart contract.)
- Click “Create Token” (The ERC-20 generator helps you to compile the smart contract into bytecode and deploy on Ethereum network.)
- Pay gas fee by ETH. (Every time you make transactions include deploying contract on Ethereum blockchain network, you need to pay the gas fee, it will go to the miners or nodes who support the network.)
- You can get transaction id address and token address. (Any transaction on Ethereum will get a transaction id address, people can check the detail of the transaction by the address on Etherscan or other Ethereum blockchain browser. For smart contract deployment, you can get a smart contract address, people can check is the contract remains the same with the original one.)
- You can add your token on your wallet. (Unlike third payment applications include Paypal or Alipay … etc. You can use any blockchain wallet that supports ERC-20. Since blockchain is a decentralized network, all token and transaction data are public to everyone, anyone can make their own application to access the data, and no single one can mute data. )
The ERC-20 generator provides only a simple version for your own token. If you like to have more functions or to interact with your own DApp, you still need to learn how to write a smart contracts and deploy on a blockchain.
Issue your own token by writing smart contract
OpenZeppelin package some useful smart contracts, we don’t need to write from scratch.
Let’s check ERC-20 smart contract on
https://docs.openzeppelin.com/contracts/2.x/erc20
and
https://github.com/OpenZeppelin/openzeppelin-contracts/tree/master/contracts/token/ERC20
Let’s rewrite the smart contract.
pragma solidity ^0.6.0;
import "https://github.com/OpenZeppelin/openzeppelin-contracts/blob/master/contracts/token/ERC20/ERC20.sol";
import "https://github.com/OpenZeppelin/openzeppelin-contracts/blob/master/contracts/token/ERC20/ERC20Detailed.sol";
contract MyToken is ERC20, ERC20Detailed {
uint256 public constant INITIAL_SUPPLY = 1e12 * (1e18);
constructor() public ERC20Detailed("TokenName", "BRIEF", 18) {
_mint(msg.sender, INITIAL_SUPPLY);
}
}
Check Github files if you like to have more functions.
You can set “TokenName”, “BRIEF” you like. Also, “INITIAL_SUPPLY” = [1e(“Token Cap Digit”)*1e(“Token Decimals”) ]. (We set “Token Cap Digit” to 12 if we want 1,000,000,000,000 tokens. mostly, people set “Token Decimals” to 18.)
Copy the contract into an online IDE. (General speaking, you need to run a Ethereum node if you want to deploy your contract, we can do it by online IDE. Remix help to run the node for you.)
Write smart contract
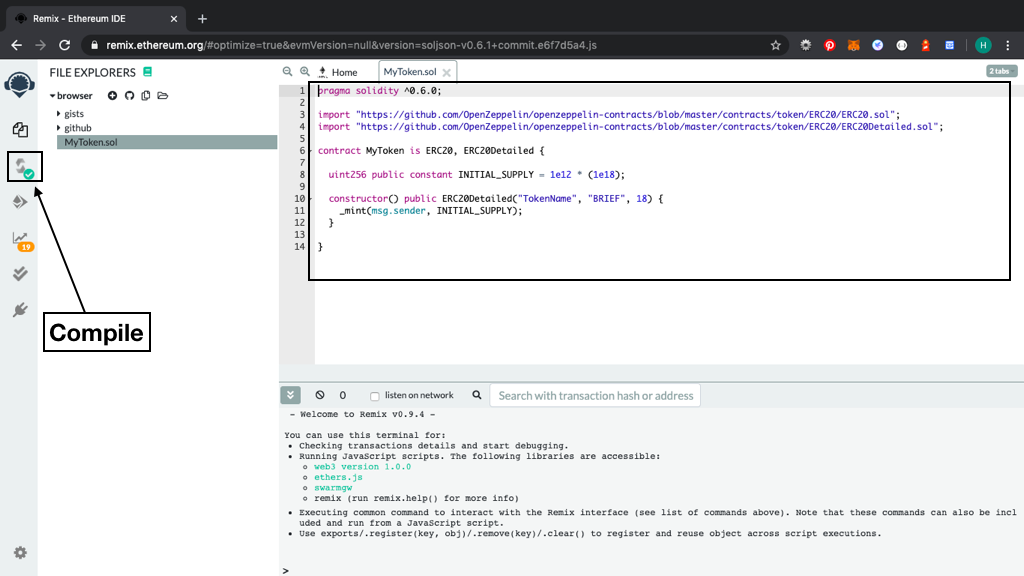
Compile smart contract into bytecode
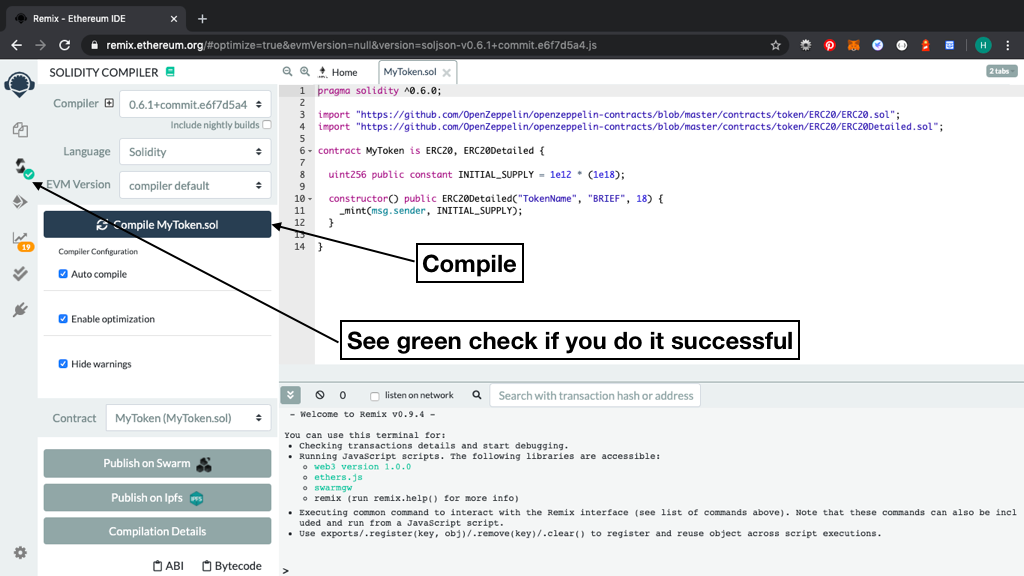
Deploy on the Ethereum blockchain network
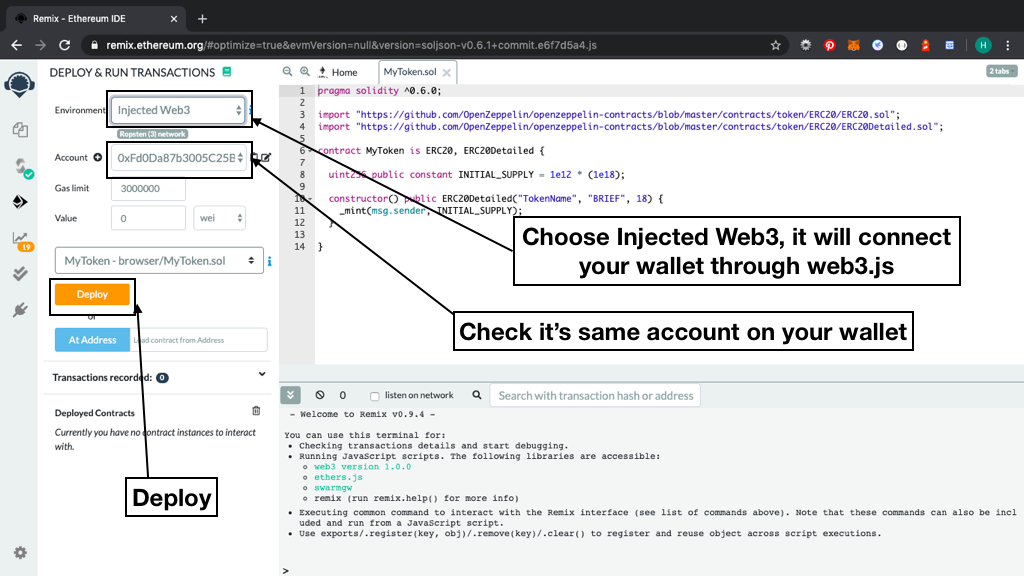
Pay gas fee
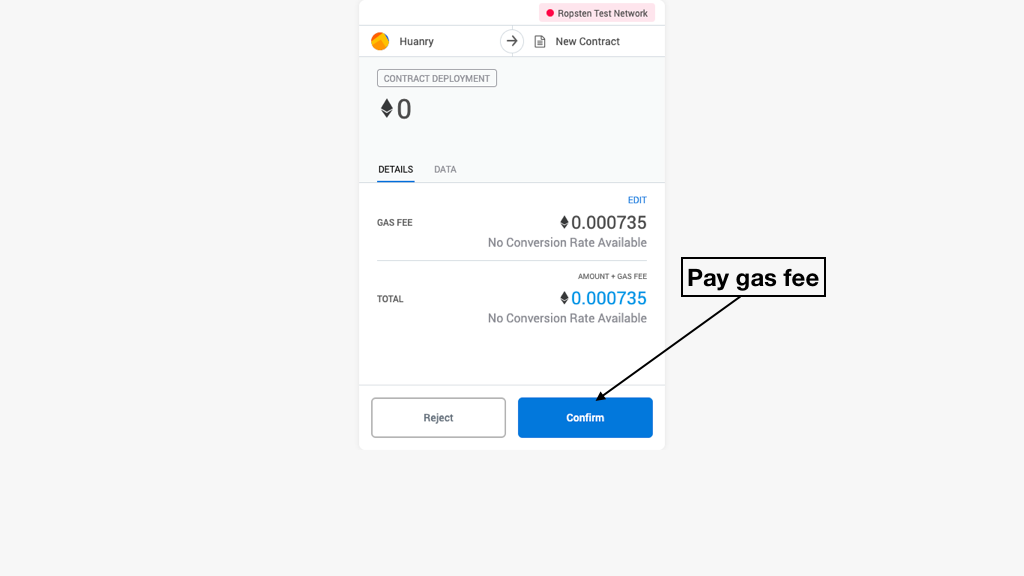
Done
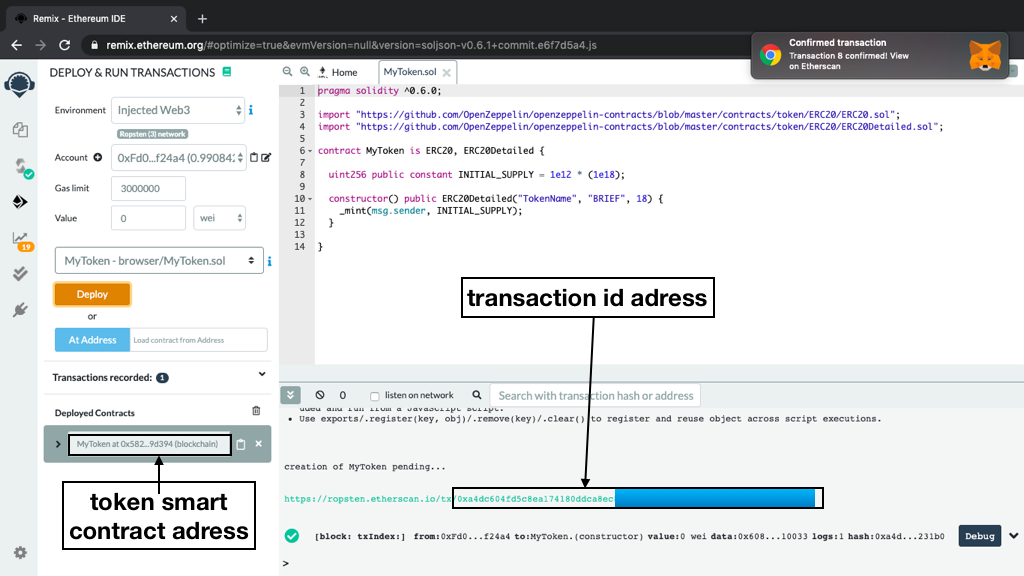
Add your own token on your wallet
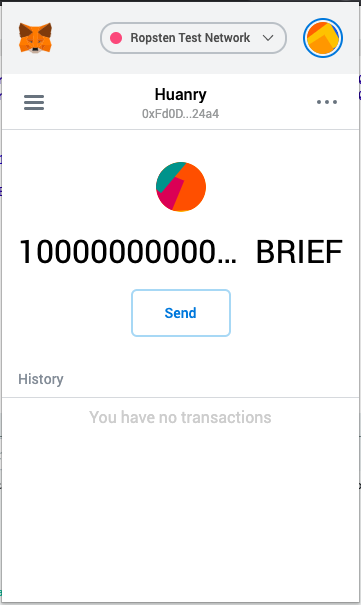
You can also check code on OpenZeppelin. The other thing you need to learn is how to interact with your own DApp with your own token.
Also, you can do all the above on main-net for the real world, the difference is you need to buy ETH or mint by your self.
Read More
Ethereum Dapp for Beginners – Full Stack Hello World Voting Ethereum Dapp Tutorial
Books for Ethereum Development on Amazon
I am new to issuing tokens. This is one of two good tutorials. I will try out both tomorrow.
Great! Leave questions if you face any problem.
I am regular visitor, how are you everybody? This article posted at this web site is actually
nice.
This is my first time visit at here and i am actually pleassant to read all at single place.
Hello, I enjoy reading all of your post. I wanted to write
a little comment to support you.
Hello! I could have sworn I’ve been to this blog before but after reading through some of the post I realized it’s new to me.
Anyways, I’m definitely delighted I found it and I’ll be bookmarking and checking
back frequently!
Excellent post! We are linking to this particularly great post on our site.
Keep up the great writing.
Hurrah, that’s what I was searching for, what a data! existing here at this website, thanks
admin of this web site.
The Theme is Blogrid By themeeverest.
Neat blog! Is your theme custom made or did you download it from somewhere?
A design like yours with a few simple adjustements would really make my blog stand
out. Please let me know where you got your theme.
Appreciate it
It’s awesome to go to see this web page and reading the views of all friends on the topic of this paragraph, while I am also eager of getting
knowledge.
Hey there! I’ve been following your weblog for some time
now and finally got the courage to go ahead and give you a shout out from Lubbock Texas!
Just wanted to mention keep up the fantastic job!
I always used to study article in news papers
but now as I am a user of internet therefore from now I am using net for articles or reviews, thanks to web.
Since the admin of this web page is working, no uncertainty very
shortly it will be famous, due to its quality
contents.
Hey very nice website!! Guy .. Beautiful .. Wonderful ..
I will bookmark your blog and take the feeds also?
I am happy to seek out a lot of helpful info here in the publish, we’d like develop more techniques on this
regard, thanks for sharing. . . . . .
Magnificent beat ! I would like to apprentice while you amend your website, how can i subscribe for a
blog web site? The account helped me a acceptable deal.
I had been tiny bit acquainted of this your broadcast offered bright clear idea
After looking into a handful of the blog posts on your
website, I really like your technique of writing a blog.
I bookmarked it to my bookmark site list and will be
checking back in the near future. Please check out my web site too and tell me how you feel.